ZenWave SDK
ZenWave SDK Helps you Create Software Easy to Understand
Note: Starting with version 2.0.0, the Maven
groupId
has changed toio.zenwave360
. The code remains fully compatible.
The heart of software is its ability to solve domain-related problems for its users. The best software supports elegant solutions to real-world problems - Eric Evans in Domain Driven Design
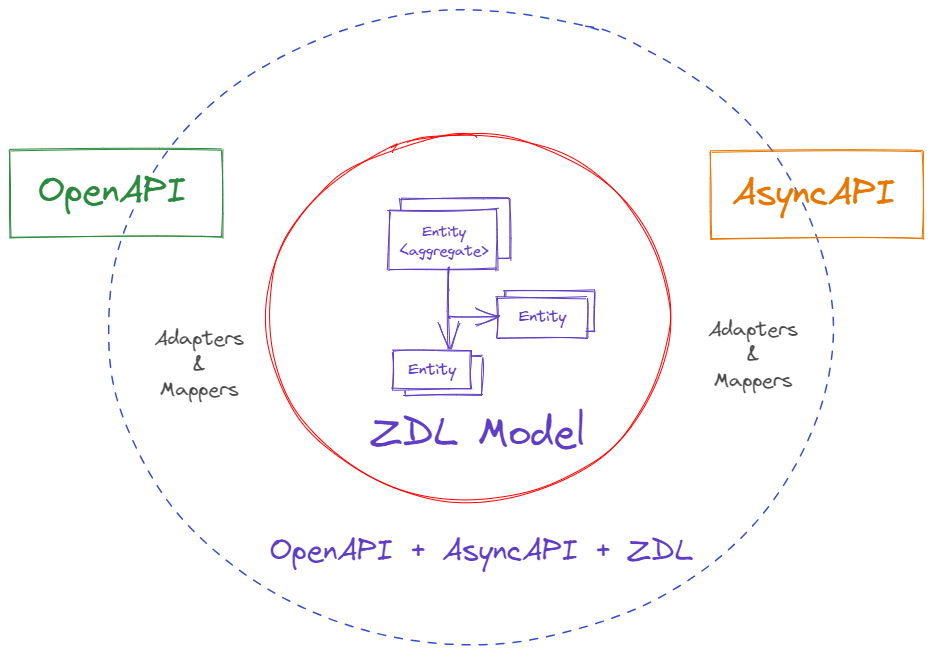
ZenWave SDK is a set of tools to convert your Domain Models into working software and tests.
It is designed to be modular, configurable and extensible. It uses the standard java classpath as loading mechanism, so you can extend its functionality adding your own custom plugins. Visit ZenWave SDK for all configuration options.
Based on Domain Driven Design (DDD) and API-First principles for Event Driven Microservices.
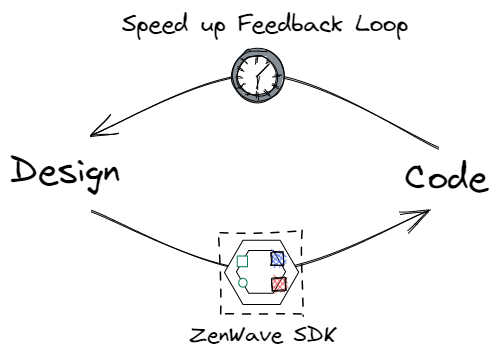
ZenWave SDK can generate code from a mix of different models including:
- ZDL Domain Language as Ubiquitous Language. You can describe the core of your Bounded Context, as well as how it connects to external systems through different adapters and APIs.
- AsyncAPI: Industry de-facto standard to describe Event-Driven Architectures for Message-based APIs.
- OpenAPI: Industry standard for Request-Response Architectures with REST APIs.
Using ZenWave Domain Language as Ubiquitous Language for modeling and describing Bounded Contexts: aggregates, entities with their relationships, services, value objects, commands and events.
ZenWave Domain Language started as an extended subset of JHipster Domain Language (JDL) that let you describe your entities and relationships.
Using ZDL Domain Language as Ubiquitous Language for Data on the Inside and API-First specs like AsyncAPI and OpenAPI to describe Inter Process Communications (IPC) for Data on the Outside.
- ZenWave Domain Language (ZDL) as Ubiquitous Language: To describe your domain core domain model.
- API-First specs like AsyncAPI and OpenAPI: to describe Inter Process Communications (IPC) between bounded contexts/microservices.
- ZenWave SDK: to generate (a lot of) infrastructure, functional and testing code from your models and APIs.
ZenWave SDK is designed to be easily extensible and adaptable to your project or your organization needs and likes.
ZenWave SDK Installation
Command Line Interface (CLI)
You can install the latest release using jbang running the following command:
jbang alias add --force --fresh --name=zw release@zenwave360/zenwave-sdkjbang zw --help list
Will output a list of all available plugins:
$ jbang zw -h listUsage: <main class> [-f] [-h[=<helpFormat>]] [-p[=<pluginClass>]][<String=Object>...][<String=Object>...]-f, --force Force overwrite-h, --help[=<helpFormat>] Help with output format-p, --plugin[=<pluginClass>]Plugin Class or short-codeINFO Reflections - Reflections took 566 ms to scan 59 urls, producing 2513 keys and 13329 valuesZW> SDK (2.0.0)Available plugins:BackendApplicationDefaultPlugin: Generates a full backend application using the provided 'layout' property (2.0.0)ZDLToOpenAPIPlugin: Generates a draft OpenAPI definitions from your ZDL entities and services. (2.0.0)ZDLToAsyncAPIPlugin: Generates a draft AsyncAPI file with events from your ZDL services. (2.0.0)OpenAPIControllersPlugin: Generates implementations based on ZDL models and OpenAPI definitions SpringMVC generated OpenAPI interfaces. (2.0.0)AsyncApiJsonSchema2PojoPlugin: Generate Plain Old Java Objects from OpenAPI/AsyncAPI schemas or full JSON-Schema files. (2.0.0)SpringCloudStreams3Plugin: Generates strongly typed SpringCloudStreams3 producer/consumer classes for AsyncAPI (2.0.0)SpringCloudStreams3AdaptersPlugin: Generates Spring Cloud Streams Consumers from AsyncAPI definitions. (2.0.0)SpringWebTestClientPlugin: Generates test for SpringMVC or Spring WebFlux using WebTestClient based on OpenAPI specification. (2.0.0)OpenAPIKaratePlugin: Generates test for KarateDSL based on OpenAPI specification. (2.0.0)JDLToAsyncAPIPlugin: Generates a full AsyncAPI definitions for CRUD operations from JDL models (2.0.0)ZdlToJsonPlugin: Prints to StdOut ZDL Model as JSON (2.0.0)OpenAPIToJDLPlugin: Generates JDL model from OpenAPI schemas (2.0.0)ZdlToMarkdownPlugin: Generates Markdown glossary from Zdl Models (2.0.0)Use: "jbang zw -p <plugin | short-code> -h" to get help on a specific plugin
If you don't find the functionality you are looking for, you can always fork an existing, standard or custom plugin.
Please refer to ZenWave SDK for more detailed installation options.
Maven Plugin
You can run any available (standard or custom) plugin as part of your maven build using the maven plugin:
Click to see the maven plugin configuration
<plugin><groupId>io.zenwave360.sdk</groupId><artifactId>zenwave-sdk-maven-plugin</artifactId><version>${zenwave.version}</version><plugin><includeProjectClasspath>false</includeProjectClasspath><!-- default is false --><addCompileSourceRoot>true</addCompileSourceRoot><!-- default is true --><addTestCompileSourceRoot>true</addTestCompileSourceRoot><!-- default is true --></plugin><executions><!-- Add executions for each generation here: --><execution><id>generate-asyncapi</id><phase>generate-sources</phase><goals><goal>generate</goal></goals><plugin><generatorName>spring-cloud-streams3</generatorName><inputSpec>classpath:model/asyncapi.yml</inputSpec><configOptions><!-- ... --><optionName>value</optionName></configOptions></plugin></execution></executions><!-- add any sdk plugin (custom or standard) as dependency here --><dependencies><dependency><groupId>io.zenwave360.sdk.plugins</groupId><artifactId>asyncapi-spring-cloud-streams3</artifactId><version>${zenwave.version}</version></dependency><dependency><groupId>io.zenwave360.sdk.plugins</groupId><artifactId>asyncapi-jsonschema2pojo</artifactId><version>${zenwave.version}</version></dependency></dependencies></plugin>
Notice how you can read spec files from the project classpath as well as the filesystem. If you want to read a spec file from inside a project dependency remember to set <includeProjectClasspath>true</includeProjectClasspath>
.
NOTE: Remember to add any plugin you want to use as dependency.
Jump to ZenWave AsyncAPI Generator for multiple examples using the maven plugin.
ZenWave SDK Workflow
You can generate complete Event Driven Microservices using DDD and API-First principles:
π Describe your Domain Model β€³ Generate OpenAPI β€³ Generate AsyncAPI β Generate API Implementations β Generate Backend β Generate Tests and Contracts π
- Start by Modeling your Domain using the ZDL Domain Language including: entities, relationships, service commands and domain events.
- Generate a draft OpenAPI definition from the ZDL model. Edit collaboratively this OpenAPI document and then generate some more functional code and tests from that definition.
- Generate a draft AsyncAPI definition for consuming async request commands and publishing domain events. Now use zenwave maven plugin to generate strongly typed business interfaces implementing some Enterprise Integration Patterns like: transactional outbox, business dead letter queue...
- Generate a complete Backend Application from your Domain Definition Model.
- Connect (by hand) your Backend Application to other systems using the generated OpenAPI and AsyncAPI definitions.
- Generate E2E, Integration tests and Consumer Contracts for the public APIs you just produced.
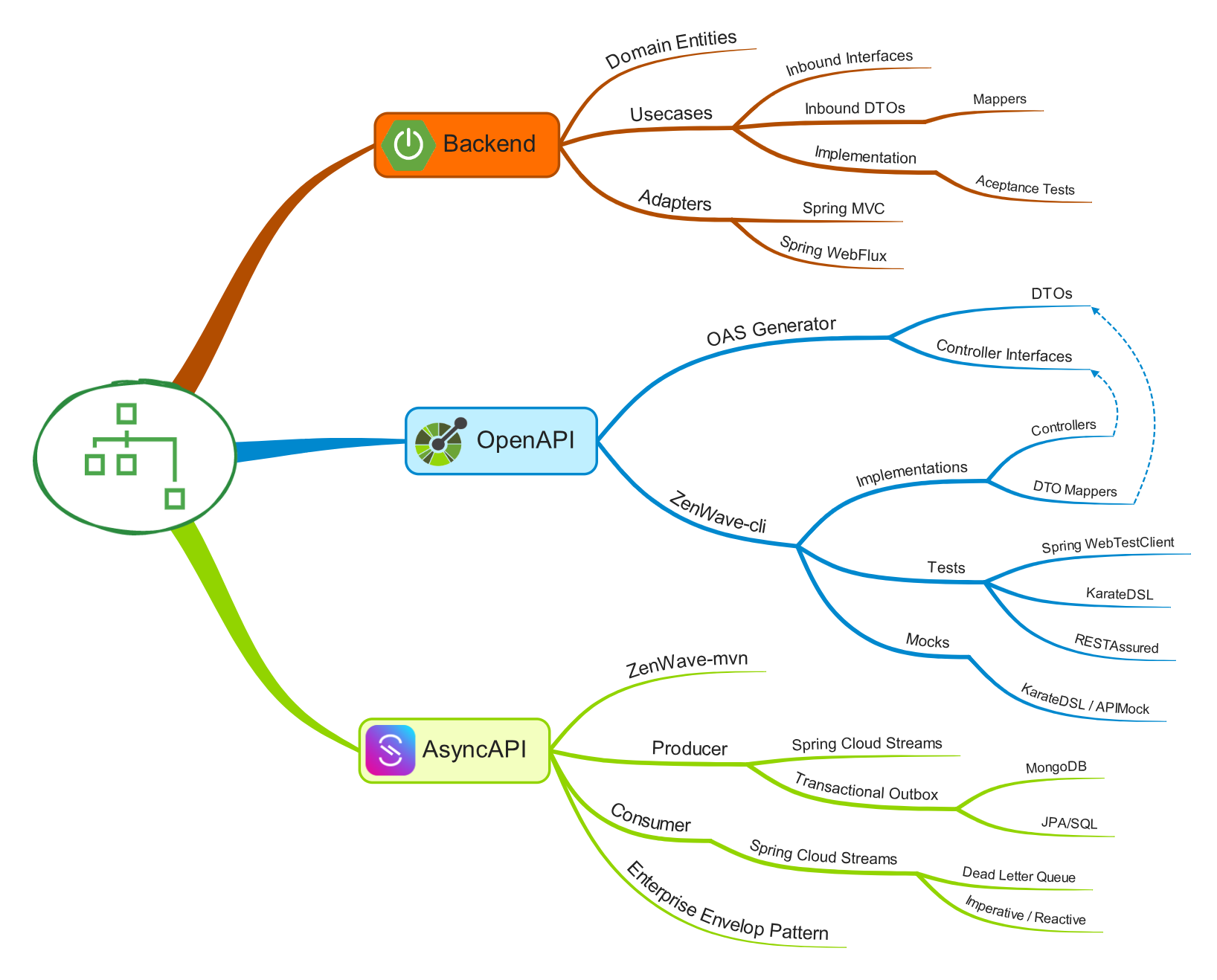
ZenWave SDK Code Generator Features (expand to see)
- JDL Backend Application (flexible hexagonal architecture)
- Domain Entities,
- Inbound
- Service Ports, DTOs, Mappers
- Implementation for CRUD operations
- Acceptance Tests: SpringData InMemory Repositories
- Outbound: SpringData Repositories, ElasticSearch... (for REST or Async see other plugins)
- Adapters:
- Spring MVC
-
Spring WebFlux
- Flavors
- MongoDB
- Imperative
-
Reactive
- JPA
- Imperative
-
Reactive
- MongoDB
- Unit/Integration Testing
- Edge Integration Testing: partial spring-boot context for outbound adapters (with testcontainers)
- Sociable Vertical Testing: manual dependency setup with in memory infrastructure test-doubles
- Vertical Integration Testing: full spring-boot context for inbound adapters (with testcontainers)
- JDL OpenAPI Controllers
- OpenAPI to Spring WebTestClient
- AsyncAPI Spring Cloud Streams3
- Consumer and Producer. Imperative and Reactive.
- Business Exceptions Dead Letter Queues Routing
- Producer with Transactional Outbox pattern
- For MongoDB
- For JDBC
- Enterprise Envelop Pattern
- Automatically fill headers at runtime from payload paths, tracing-id supplier...
- Consumer and Producer. Imperative and Reactive.
- JDL to Specs
- JDL to OpenAPI
- JDL to AsyncAPI
- AsyncAPI schemas
- AVRO schemas
- API Testing
- KarateDSL
- OpenAPI to Karate E2E Tests (please use KarateIDE VSCode Extension instead)
- OpenAPI to Karate/ApiMock Stateful Mocks (please use KarateIDE VSCode Extension and ZenWave ApiMock instead)
- OpenAPI to Spring WebTestClient
- OpenAPI to REST-assured
-
OpenAPI to Pact (postponed sine die)
- KarateDSL
- Reverser Engineering
- OpenAPI 2 JDL
- Java 2 JDL
- Spring Data MongoDB annotations
- JPA annotations
Generated Code Structure
Generated code follows a flexible onion/hexagonal architecture. Separating core, inbound, implementation and outbound form infrastructure and adapters.
Core domain entities and aggregates are annotated for persistence with JPA or SpringData/MongoDB annotations, avoiding unnecessary translation layers (mappers and dtos).
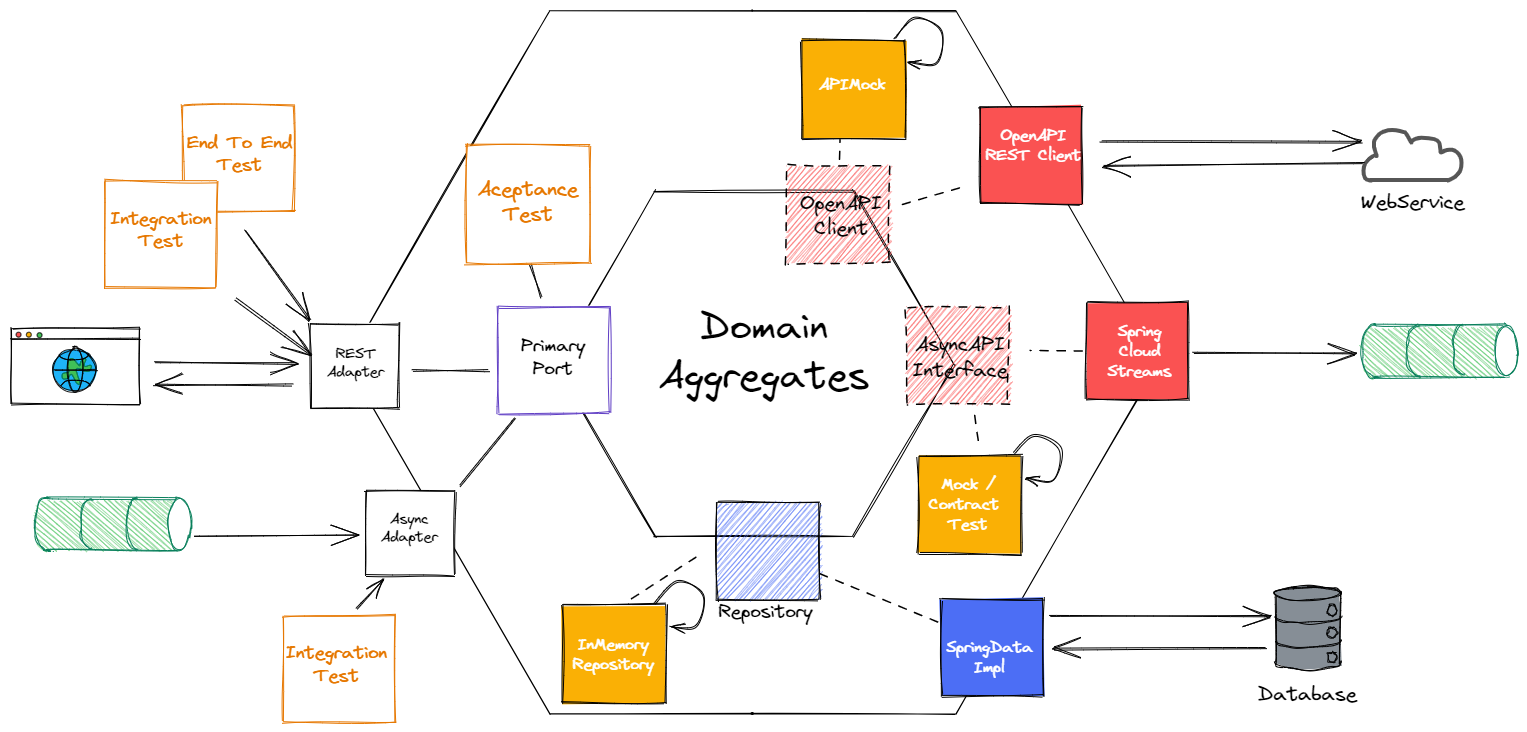
Project structure:
π¦ <basePackage>π¦ adaptersββ web| ββ RestControllers (spring mvc)ββ eventsββ *EventListeners (spring-cloud-streams)π¦ coreββ π¦ domain| ββ (entities and aggregates)ββ π¦ inbound| ββ dtos/| ββ ServiceInterface (inbound service interface)ββ π¦ outbound| ββ mongodb| | ββ *RepositoryInterface (spring-data interface)| ββ jpa| ββ *RepositoryInterface (spring-data interface)ββ π¦ implementationββ mappers/ββ ServiceImplementation (inbound service implementation)π¦ infrastructureββ mongodb| ββ CustomRepositoryImpl (spring-data custom implementation)ββ jpaββ CustomRepositoryImpl (spring-data custom implementation)
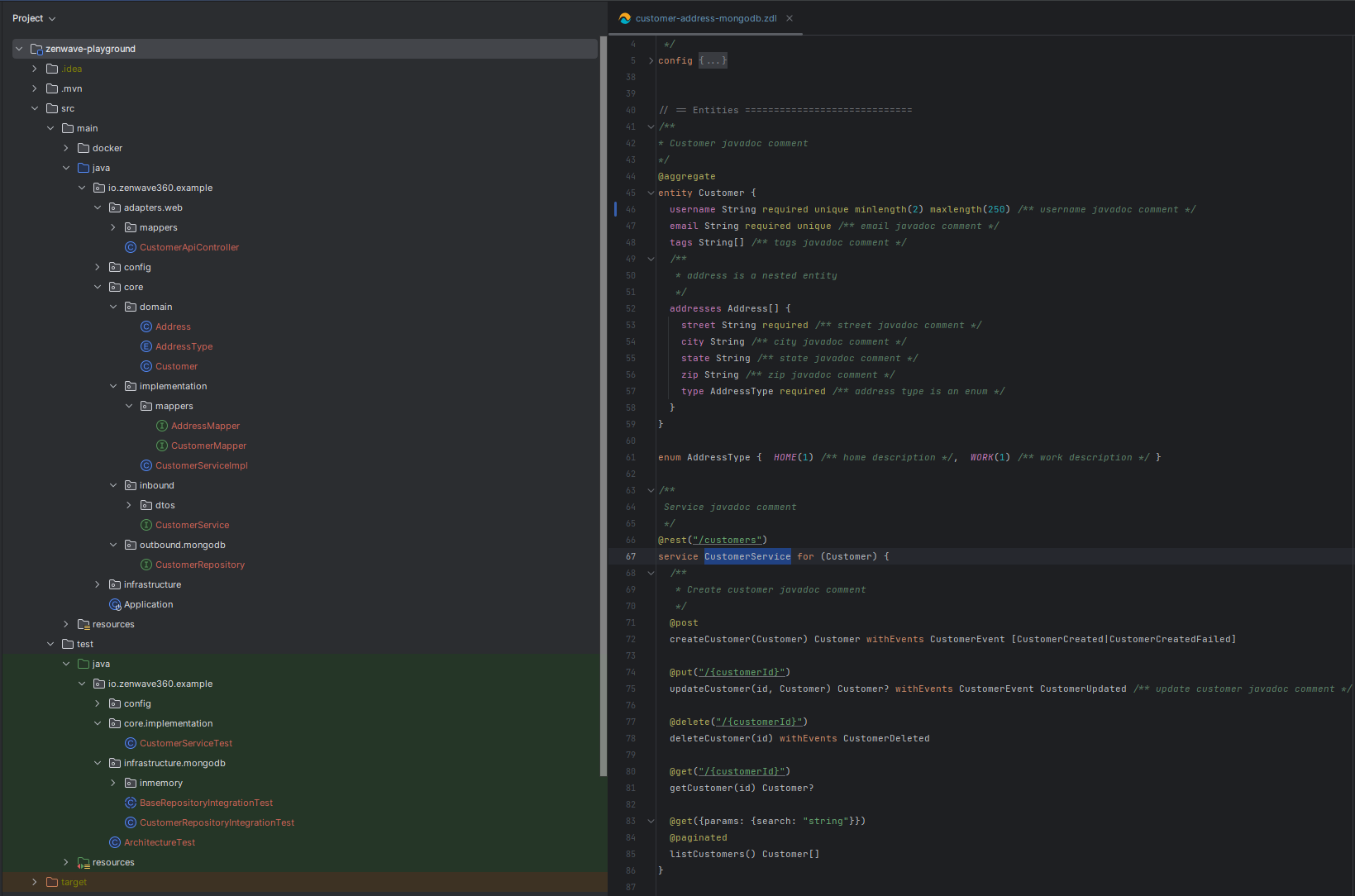